Svelte Carousel - Flowbite
Use the carousel component to slide through multiple elements and images using custom controls, indicators, intervals, and options
The carousel component can be used to cycle through a set of elements using custom options, controls, and indicators.
Setup #
- Svelte
<script>
import { Carousel, CarouselTransition } from 'flowbite-svelte';
// ./imageData/+server.js has the following
export const images = [
{
id: 0,
name: 'Cosmic timetraveler',
imgurl: '/images/carousel/cosmic-timetraveler-pYyOZ8q7AII-unsplash.webp',
attribution: 'cosmic-timetraveler-pYyOZ8q7AII-unsplash.com'
},
{
id: 1,
name: 'Cristina Gottardi',
imgurl: '/images/carousel/cristina-gottardi-CSpjU6hYo_0-unsplash.webp',
attribution: 'cristina-gottardi-CSpjU6hYo_0-unsplash.com'
},
{
id: 2,
name: 'Johannes Plenio',
imgurl: '/images/carousel/johannes-plenio-RwHv7LgeC7s-unsplash.webp',
attribution: 'johannes-plenio-RwHv7LgeC7s-unsplash.com'
},
{
id: 3,
name: 'Jonatan Pie',
imgurl: '/images/carousel/jonatan-pie-3l3RwQdHRHg-unsplash.webp',
attribution: 'jonatan-pie-3l3RwQdHRHg-unsplash.com'
},
{
id: 4,
name: 'Mark Harpur',
imgurl: '/images/carousel/mark-harpur-K2s_YE031CA-unsplash.webp',
attribution: 'mark-harpur-K2s_YE031CA-unsplash'
},
{
id: 5,
name: 'Pietro De Grandi',
imgurl: '/images/carousel/pietro-de-grandi-T7K4aEPoGGk-unsplash.webp',
attribution: 'pietro-de-grandi-T7K4aEPoGGk-unsplash'
},
{
id: 6,
name: 'Sergey Pesterev',
imgurl: '/images/carousel/sergey-pesterev-tMvuB9se2uQ-unsplash.webp',
attribution: 'sergey-pesterev-tMvuB9se2uQ-unsplash'
},
{
id: 7,
name: 'Solo travel goals',
imgurl: '/images/carousel/solotravelgoals-7kLufxYoqWk-unsplash.webp',
attribution: 'solotravelgoals-7kLufxYoqWk-unsplash'
}
];
</script>
Default Carousel #
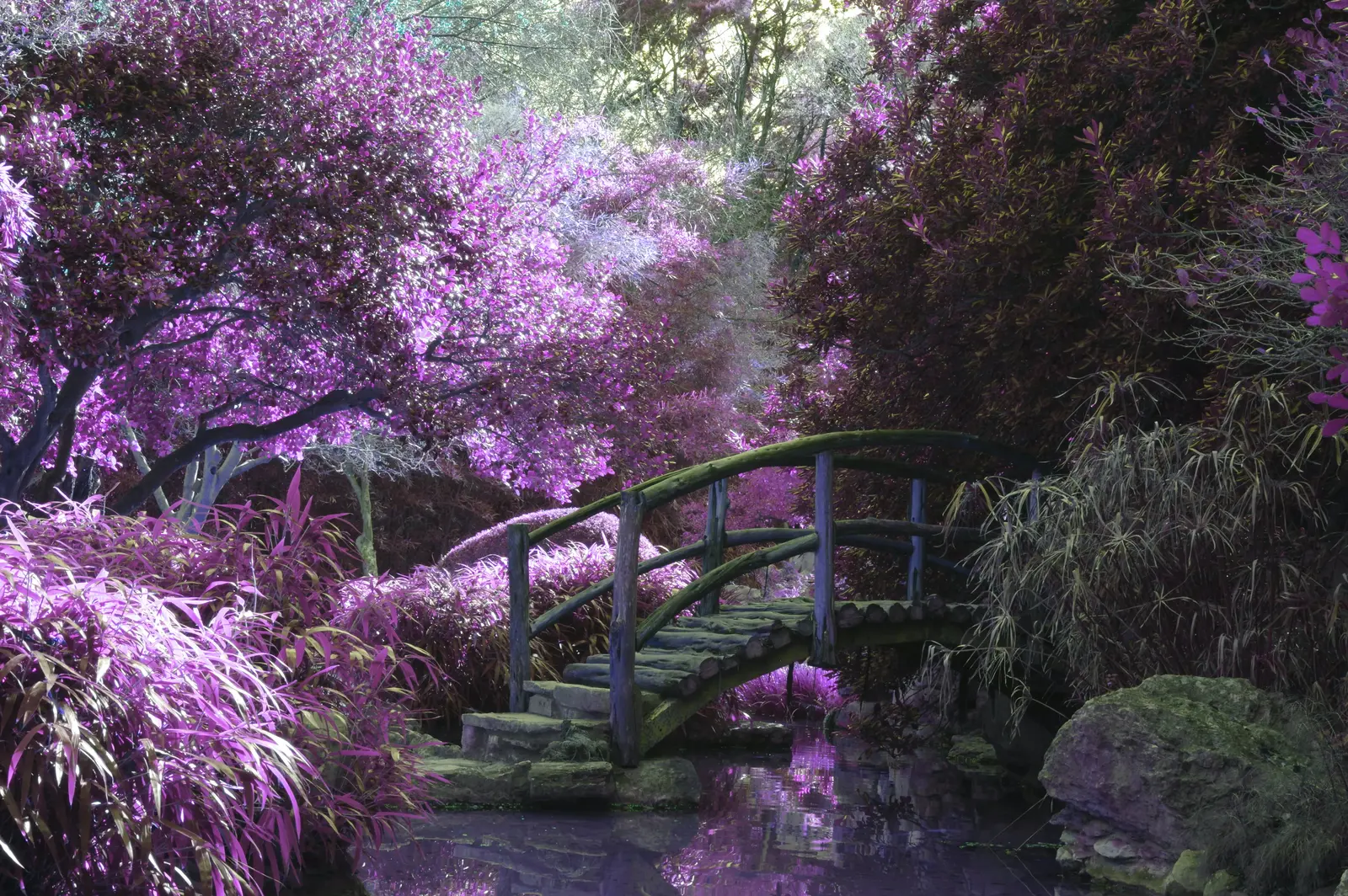
Cosmic timetraveler
- Svelte
<script>
import { Carousel } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
</script>
<div class="max-w-4xl">
<Carousel {images} />
</div>
Loop #
Use `loop` prop to loop the carousel. Use `duration=number` to set the interval
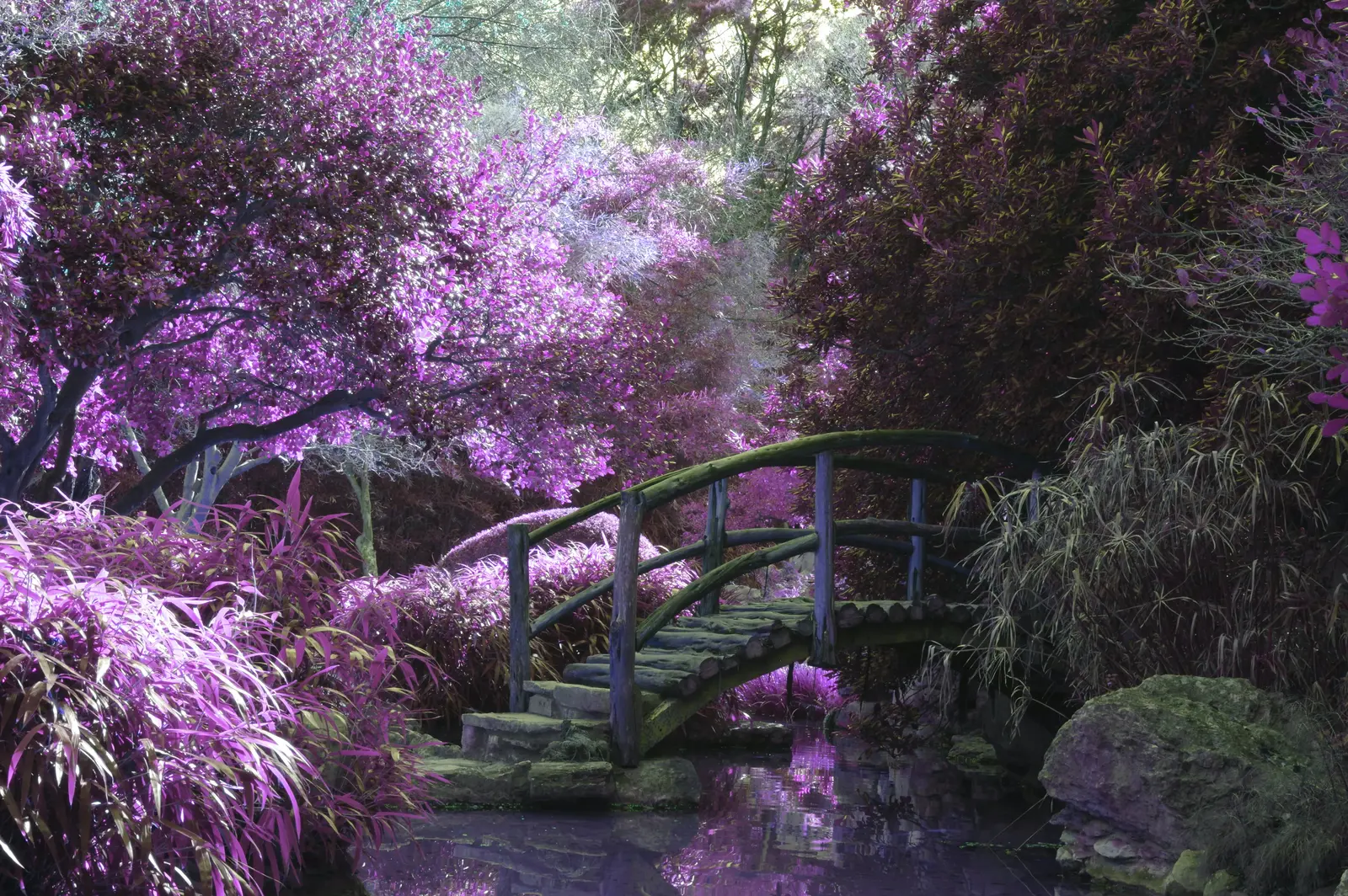
- Svelte
<script>
import { Carousel } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
let showThumbs = false;
let showCaptions = false;
</script>
<div class="max-w-4xl">
<Carousel {images} loop {showCaptions} {showThumbs} duration="3000" />
</div>
Without thumbnails #
The `showThumbs` is set to `true`. Set it to `false` to hide it.
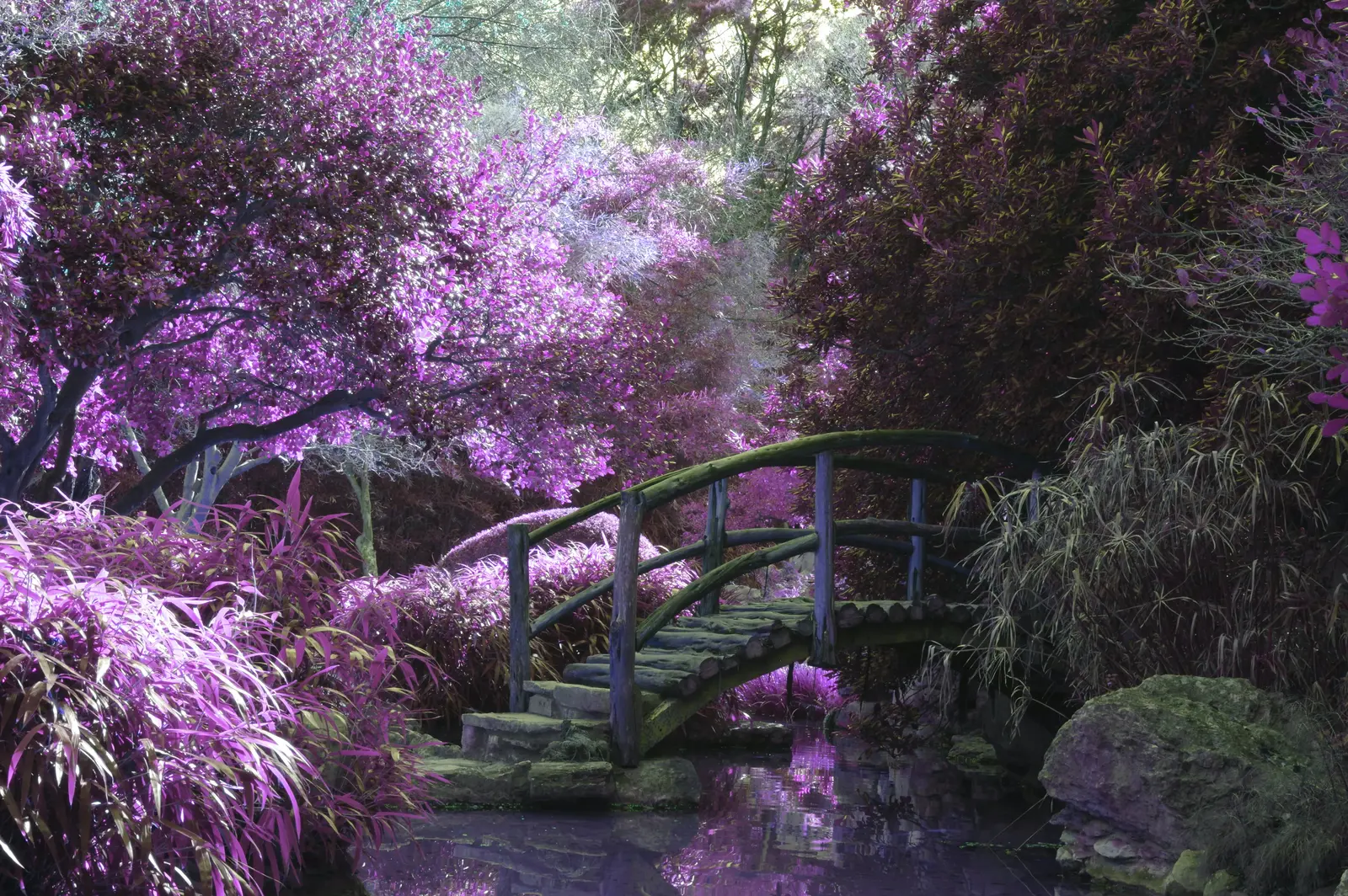
Cosmic timetraveler
- Svelte
<script>
import { Carousel } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
let showThumbs = false;
</script>
<div class="max-w-4xl">
<Carousel {images} {showThumbs} />
</div>
Without caption #
To hide the caption, set `showCaptions` to `false`.
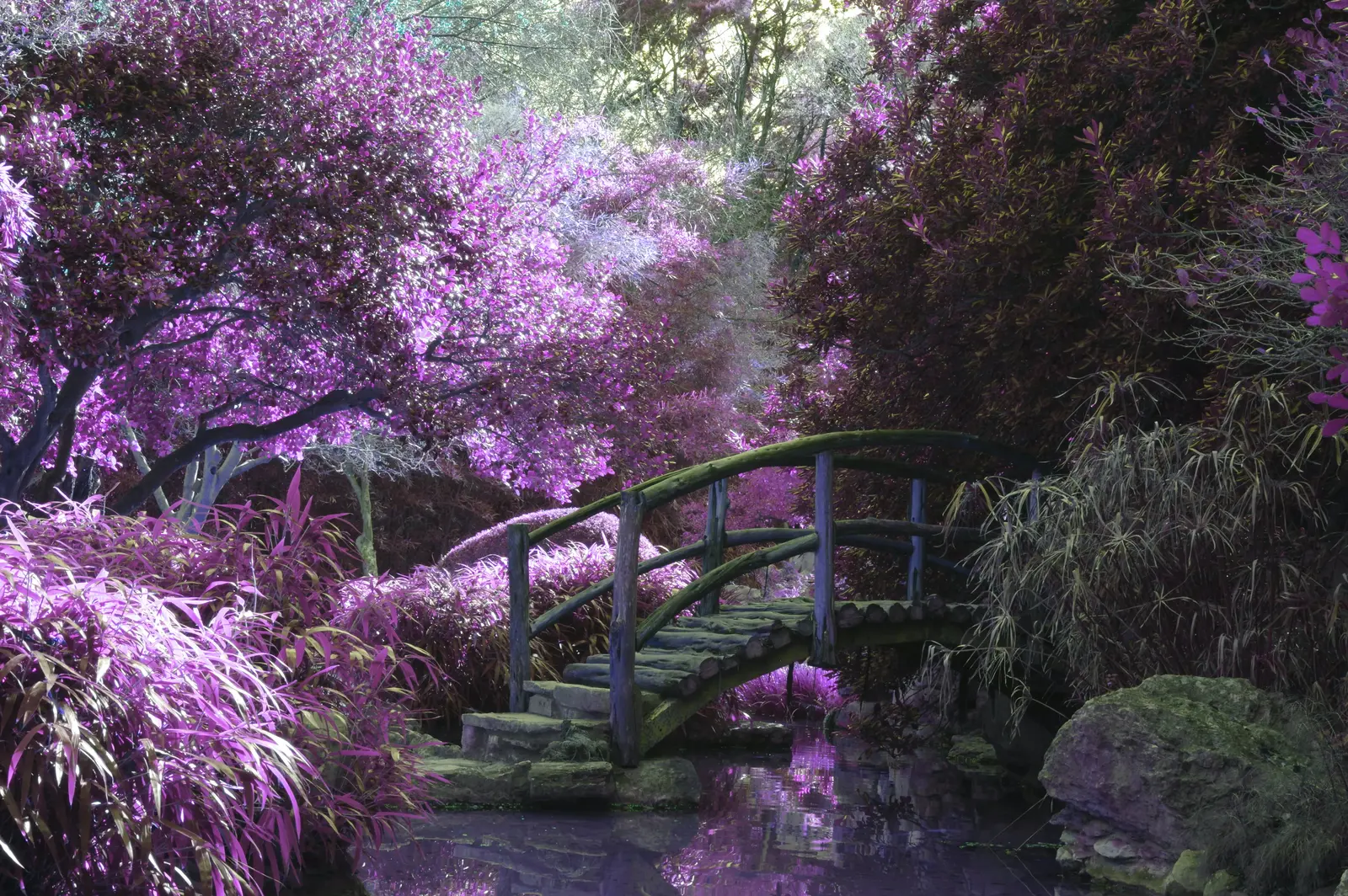
- Svelte
<script>
import { Carousel } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
let showThumbs = false;
let showCaptions = false;
</script>
<div class="max-w-4xl">
<Carousel {images} {showThumbs} {showCaptions} />
</div>
Without indicators #
To hide the indicators, set `showIndicators` to `false`.
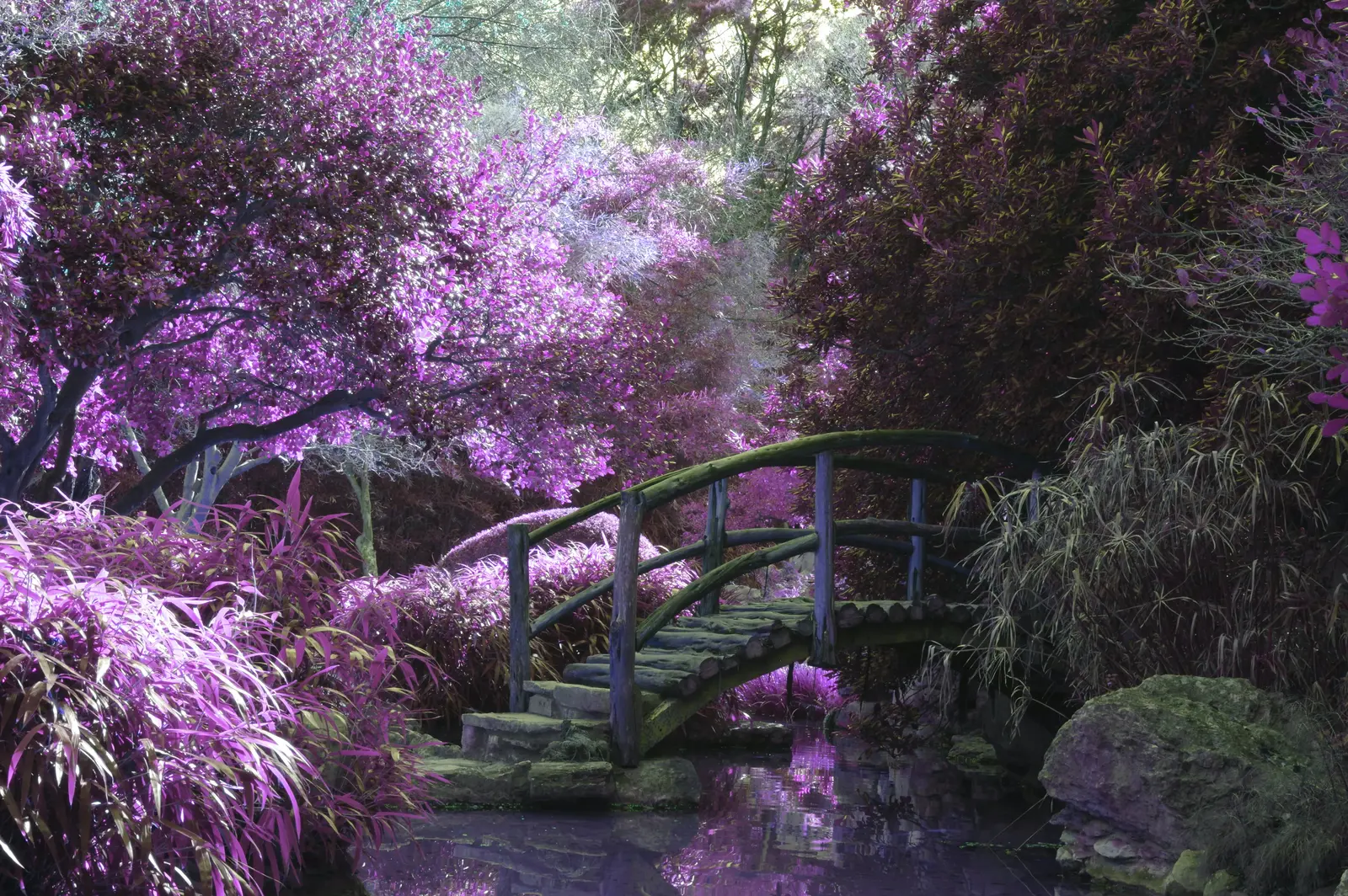
- Svelte
<script>
import { Carousel } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
let showThumbs = false;
let showCaptions = false;
let showIndicators = false;
</script>
<div class="max-w-4xl">
<Carousel {images} {showThumbs} {showCaptions} {showIndicators} />
</div>
Without slide controllers #
To hide the slide controllers, set `slideControls` to `false`.
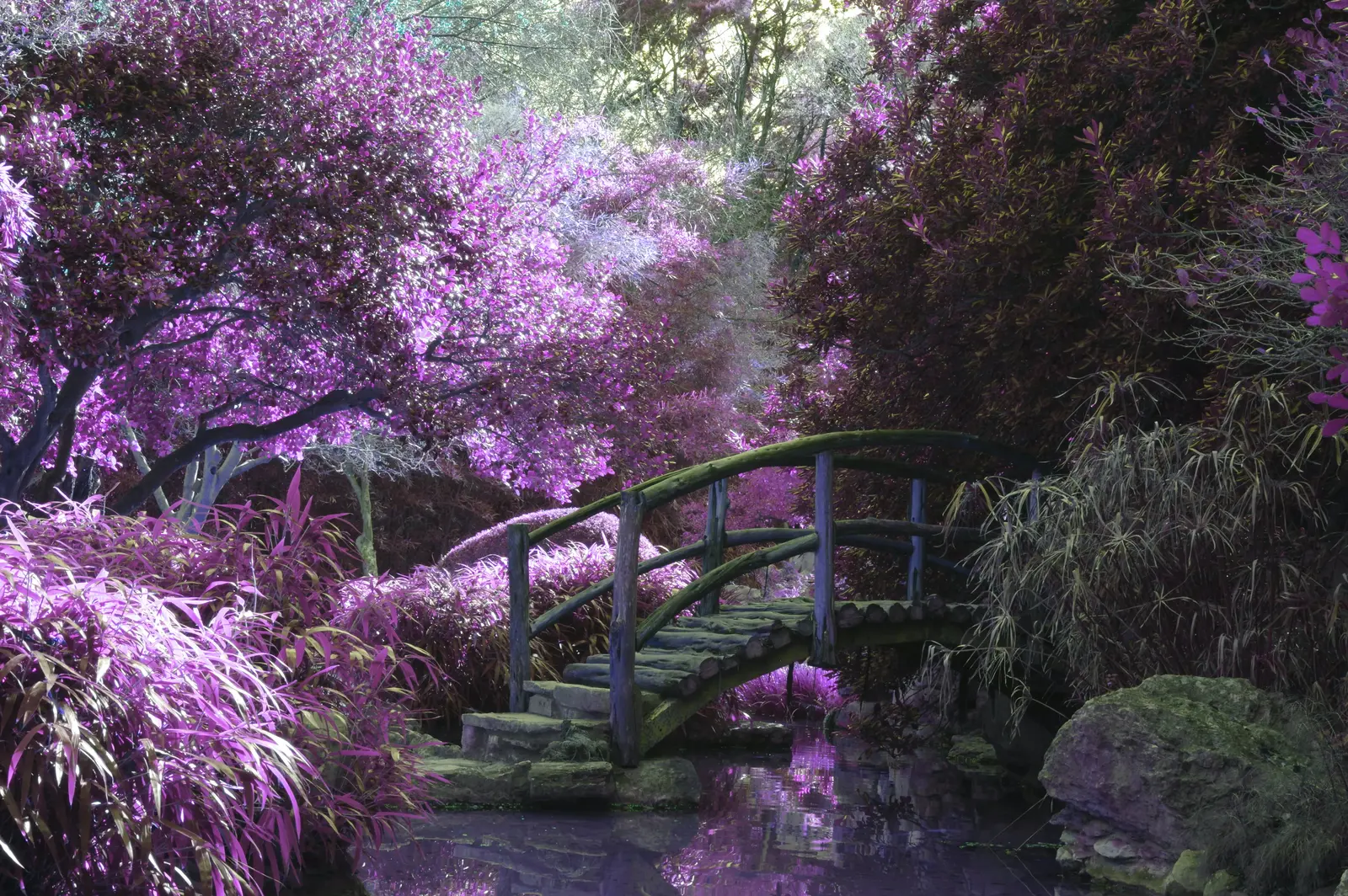
- Svelte
<script>
import { Carousel } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
let showThumbs = false;
let showCaptions = false;
let slideControls = false;
</script>
<div class="max-w-4xl">
<Carousel {images} {showThumbs} {showCaptions} {slideControls} />
</div>
Carousel transition #
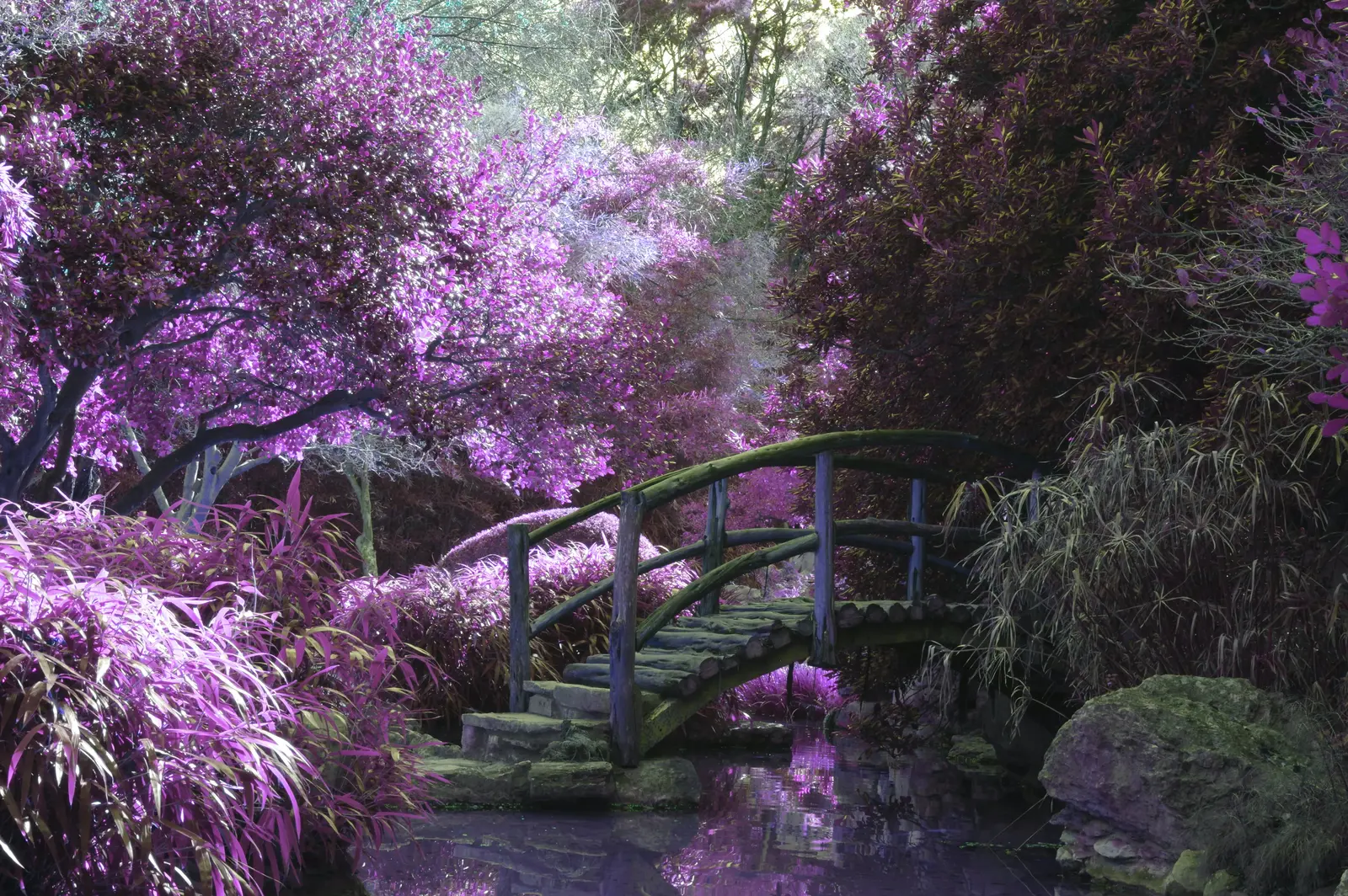
Cosmic timetraveler
- Svelte
<script>
import { CarouselTransition } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
</script>
<div class="max-w-4xl">
<CarouselTransition {images} transitionType="fade" transitionParams={{ delay: 300, duration: 500 }} />
</div>
Loop #
Use `loop` prop to loop the carousel. Use `duration=number` to set the interval
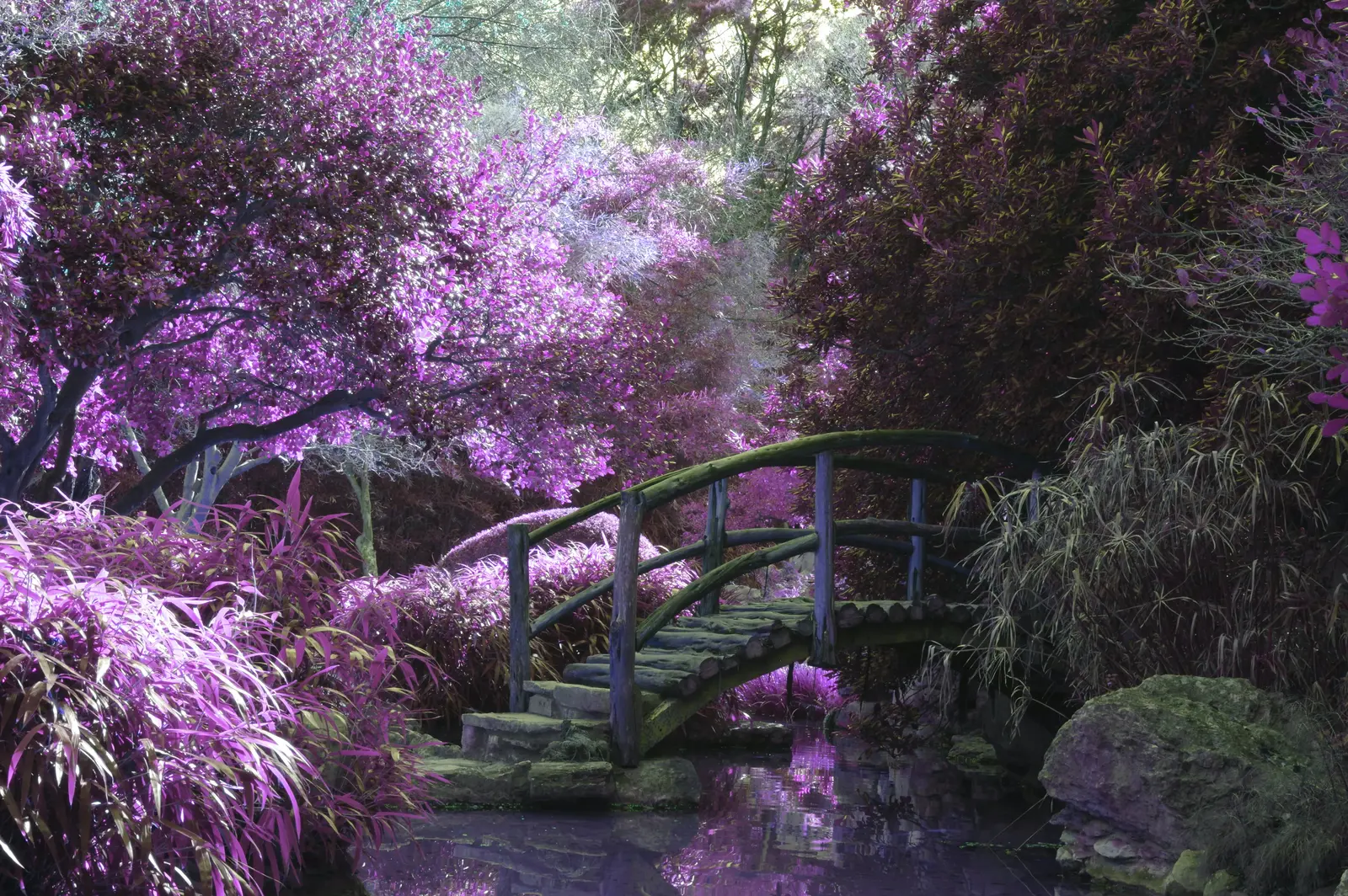
- Svelte
<script>
import { CarouselTransition } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
</script>
<div class="max-w-4xl">
<CarouselTransition {images} loop transitionType="fade" transitionParams={{ duration: 1000 }} showCaptions={false} showThumbs={false} duration="5000" />
</div>
Fly example #
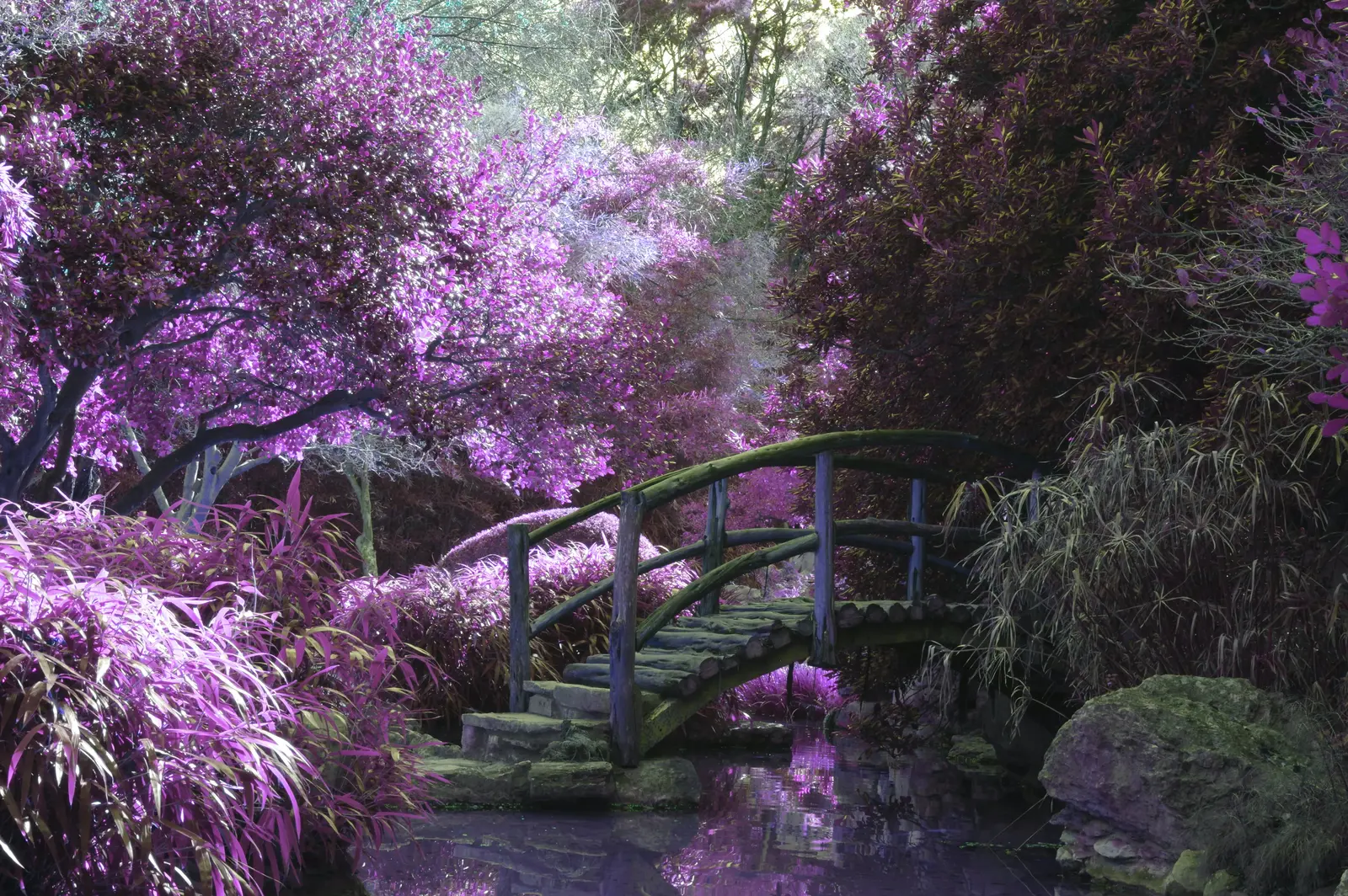
- Svelte
<script>
import { CarouselTransition } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
</script>
<div class="max-w-4xl">
<CarouselTransition {images} transitionType="fly" transitionParams={{ delay: 250, duration: 300, x: 100 }} showCaptions={false} showThumbs={false} />
</div>
Slide example #
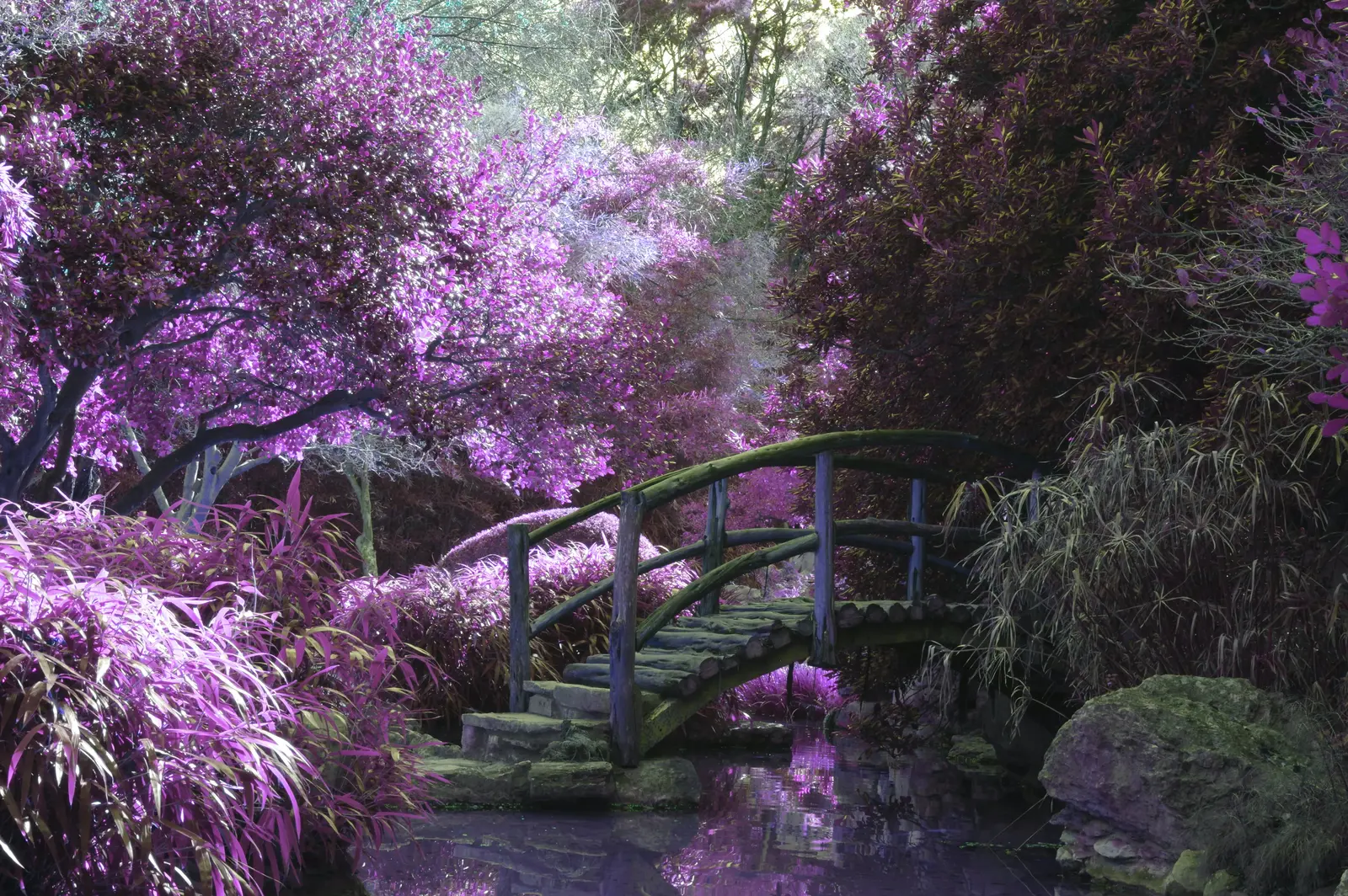
- Svelte
<script>
import { CarouselTransition } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
import { bounceInOut } from 'svelte/easing';
</script>
<div class="max-w-4xl">
<CarouselTransition {images} transitionType="slide" transitionParams={{ duration: 1500, easing: bounceInOut }} showCaptions={false} showThumbs={false} />
</div>
Custom Styling #
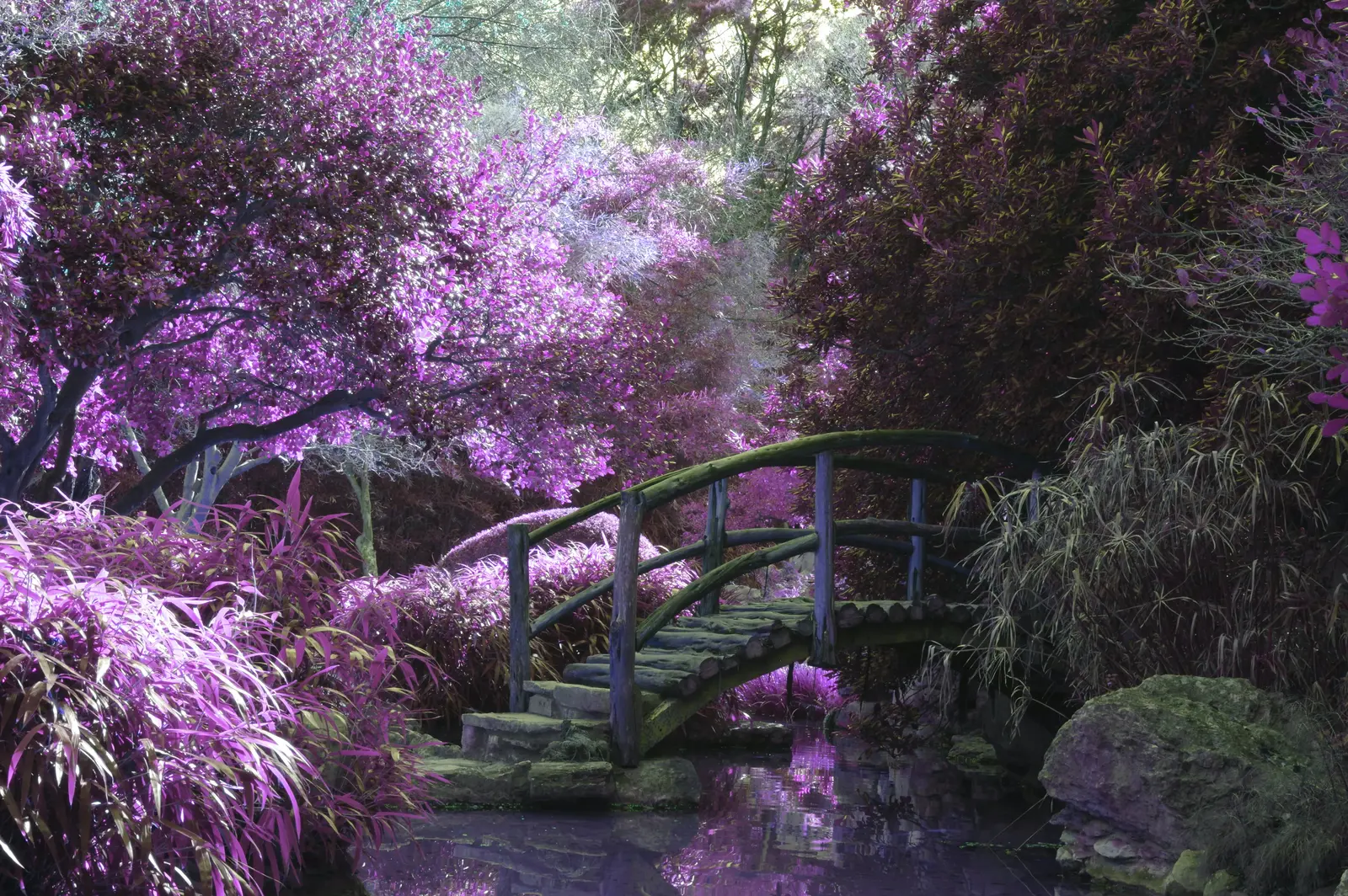
- Svelte
<script>
import { Carousel } from 'flowbite-svelte';
import { images } from './imageData/+server.js';
</script>
<div class="max-w-4xl dark p-2 bg-gray-100 dark:bg-gray-800">
<Carousel {images} showCaptions={false} classSlide="flex items-center justify-center h-full w-full !rounded-none !bg-transparent" classDiv="w-full !h-[300px] sm:!h-[400px] !rounded-none !bg-transparent" imgFit="contain" classImg="!bg-none !rounded-md animate-[fadeIn_.5s_ease-in-out_1] h-full" classThumb="p-0 rounded-md shadow-xl hover:outline hover:outline-primary-500" classThumbDiv="bg-transparent" thumbBtnClass="m-2" />
</div>
Component data #
The component has the following props, type, and default values. See types page for type information.
Carousel #
- Use the
classDiv
prop to overwritedivClass
. - Use the
classIndicatorDiv
prop to overwriteindicatorDivCls
. - Use the
classCaption
prop to overwritecaptionClass
. - Use the
classIndicator
prop to overwriteindicatorClass
.
Props
Name | Type | Default |
---|---|---|
id | string | 'default-carousel' |
showIndicators | boolean | true |
showCaptions | boolean | true |
showThumbs | boolean | true |
images | any[] | |
slideControls | boolean | true |
loop | boolean | false |
duration | number | 2000 |
divClass | string | 'overflow-hidden relative h-56 rounded-lg sm:h-64 xl:h-80 2xl:h-96' |
indicatorDivClass | string | 'flex absolute bottom-5 left-1/2 z-30 space-x-3 -translate-x-1/2' |
captionClass | string | 'h-10 bg-gray-300 dark:bg-gray-700 dark:text-white p-2 my-2 text-center' |
indicatorClass | string | 'w-3 h-3 rounded-full bg-gray-100 hover:bg-gray-300 opacity-60' |
slideClass | string | 'flex items-center justify-center h-full w-full' |
imgFit | 'cover' | 'contain' | 'fill' | 'none' | 'scale-down' | 'cover' |
imgClass | string = `object-${imgFit} ${imgFit === 'cover' && 'w-full'} h-full`; | |
thumbClass | string | 'opacity-40' |
thumbDivClass | string | 'flex flex-row justify-center bg-gray-100 w-full' |
thumbBtnClass | string | '' |
Events
Names |
---|
on:mousemove |
on:mouseup |
on:touchmove |
on:touchend |
on:mousedown |
on:touchstart |
on:click |
on:click |
on:click |
on:click |
Slots
Names |
---|
previous |
next |
CarouselTransition #
Props
Name | Type | Default |
---|---|---|
id | string | 'default-transition-carousel' |
showIndicators | boolean | true |
showCaptions | boolean | true |
showThumbs | boolean | true |
images | any[] | |
slideControls | boolean | true |
transitionType | TransitionTypes | 'fade' |
transitionParams | TransitionParamTypes | {} |
loop | boolean | false |
duration | number | 2000 |
divClass | string | 'overflow-hidden relative h-56 rounded-lg sm:h-64 xl:h-80 2xl:h-96' |
indicatorDivClass | string | 'flex absolute bottom-5 left-1/2 z-30 space-x-3 -translate-x-1/2' |
captionClass | string | 'h-10 bg-gray-300 dark:bg-gray-700 dark:text-white p-2 my-2 text-center' |
indicatorClass | string | 'w-3 h-3 rounded-full bg-gray-100 hover:bg-gray-300 opacity-60' |
slideClass | string | '' |
transitionDivClass | string | 'h-full w-full' |
imgFit | 'cover' | 'contain' | 'fill' | 'none' | 'scale-down' | 'cover' |
imgClass | string = `object-${imgFit} ${imgFit === 'cover' && 'w-full'} h-full`; | |
thumbClass | string | 'opacity-40' |
thumbDivClass | string | 'flex flex-row justify-center bg-gray-100 w-full' |
thumbBtnClass | string | '' |
Events
Names |
---|
on:multiple |
on:click |
on:click |
on:click |
on:click |
Slots
Names |
---|
previous |
next |
Caption #
Props
Name | Type | Default |
---|---|---|
caption | string | '' |
captionClass | string | '' |
pClass | string | 'text-gray-900 dark:text-white' |
Indicator #
Props
Name | Type | Default |
---|---|---|
color | IndicatorColorType | 'gray' |
rounded | boolean | false |
size | 'xs' | 'sm' | 'md' | 'lg' | 'xl' | 'md' |
border | boolean | false |
placement | PlacementType | undefined | undefined |
offset | boolean | true |
Slide #
Props
Name | Type | Default |
---|---|---|
image | string | '' |
altTag | string | '' |
attr | string | '' |
slideClass | string | '' |
imgClass | string | '' |
Thumbnail #
Props
Name | Type | Default |
---|---|---|
thumbImg | string | '' |
altTag | string | '' |
titleLink | string | '' |
id | number | |
thumbWidth | number | 100 |
selected | boolean | false |
thumbClass | string | '' |
thumbBtnClass | string | '' |
Events
Names |
---|
on:click |